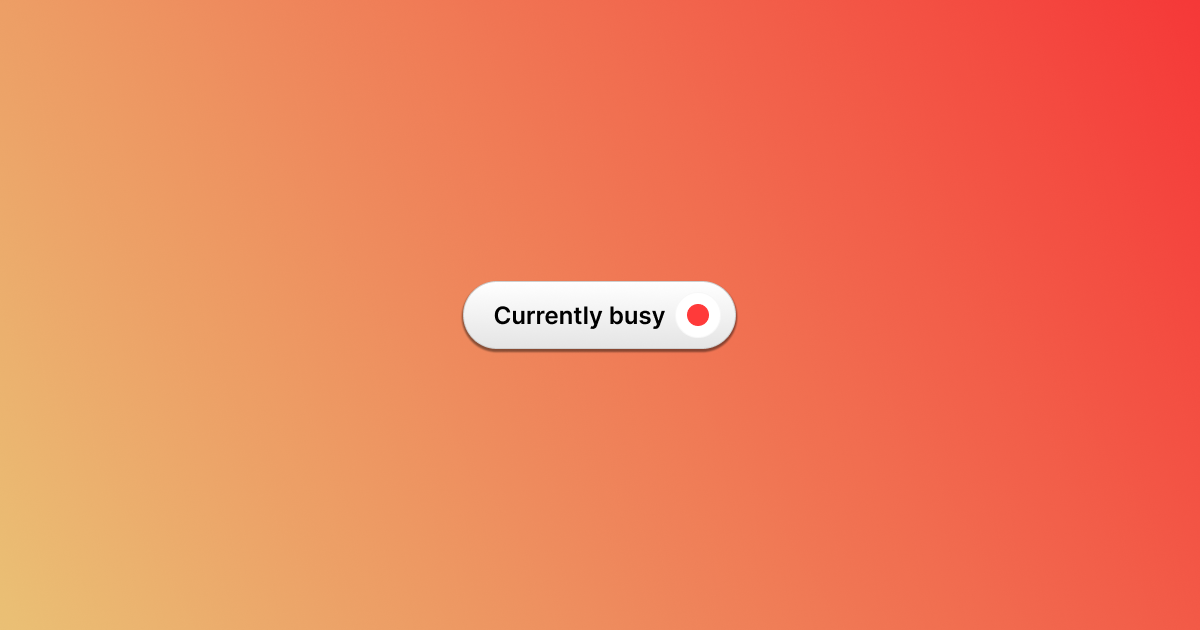
Building a Google Calendar Status Component in React
We're going to build a React component that fetches your current Google Calendar status and displays it on your website. Imagine having a "Busy" or "Free" status automatically updating on your portfolio or blog.
What You'll Need
Before we start coding, you'll need two key pieces of information, these are your Google Calendar ID and your Google API Key.
Getting your Google API Key
- Go to the Google Cloud Console.
- Create a new project or select an existing one.
- Navigate to "Credentials" and create a new API key.
- Enable the Google Calendar API for your project.
Getting your Google Calendar ID
- Open Google Calendar
- Click on the settings gear icon, then go to "Settings."
- Click on the calendar you want to use under "Settings for my calendars."
- You'll find the Calendar ID there. It usually looks like an email address.
Setting Up Your Environment
Save these keys in your .env file like so:
REACT_APP_API_KEY=YOUR_API_KEY;
REACT_APP_CALENDAR_ID=YOUR_CALENDAR_ID;
Building the React Component
Now that we've got our keys, let's build a React component that'll show if we're busy or free.
const GoogleCalendarStatus = () => {
// Here we want to store the state of busy/free &
// also fetch data from our Google Calendar API
return (
// Return UI Component
);
};
export default GoogleCalendarStatus;
Handling State and Fetching Data
First, let's create a state variable to handle our status. We'll initialize it as "Loading..." and later update it to either "Busy" or "Free."
We'll also grab our keys from the .env file and store the current time.
import React, { useState, useEffect } from 'react';
import axios from 'axios';
const GoogleCalendarStatus = () => {
const [status, setStatus] = useState('Loading...');
useEffect(() => {
const API_KEY = process.env.REACT_APP_API_KEY;
const CALENDAR_ID = process.env.REACT_APP_CALENDAR_ID;
const currentTime = new Date().toISOString();
}, []);
return (
// Return UI Component
);
};
export default GoogleCalendarStatus;
Fetching Data from Google Calendar
Finally, let's fetch our calendar data. We'll use this URL, plugging in our variables:
https://www.googleapis.com/calendar/v3/calendars/${CALENDAR_ID}/events?key=${API_KEY}&timeMin=${currentTime}&maxResults=1&orderBy=startTime&singleEvents=true
And here's how you fetch the data:
import React, { useState, useEffect } from "react";
const GoogleCalendarStatus = () => {
const [status, setStatus] = useState("Loading...");
useEffect(() => {
const API_KEY = process.env.REACT_PUBLIC_API_KEY;
const CALENDAR_ID = process.env.REACT_PUBLIC_CALENDAR_ID;
const currentTime = new Date().toISOString();
const url = `https://www.googleapis.com/calendar/v3/calendars/${CALENDAR_ID}/events?key=${API_KEY}&timeMin=${currentTime}&maxResults=1&orderBy=startTime&singleEvents=true`;
fetch(url)
.then((response) => response.json())
.then((data) => {
const events = data.items;
if (events && events.length > 0) {
setStatus("Busy");
} else {
setStatus("Free");
}
})
.catch((error) => {
console.error("Error fetching data:", error);
setStatus("Error");
});
}, []);
return (
<div>
<>Currently {status}</>
</div>
);
};
export default GoogleCalendarStatus;
This will simply display busy / free depending on our status.
Style our component
Finally, lets add some styles and colors to our component. Here I've decided to go with tailwind for simplicity but the choice of style framework is yours!
<div
className={`gap-2 inline-flex ${
status === "Busy" ? "bg-red-100" : "bg-green-100"
} text-black items-center py-2 pl-5 pr-2 rounded-full`}
>
<div className="font-medium">Currently {status}</div>
<div className="flex items-center justify-center bg-white w-8 h-8 rounded-full">
<div
className={`w-4 h-4 rounded-full ${
status === "Busy" ? "bg-red-500" : "bg-green-500"
}`}
/>
</div>
</div>
This will end up look like something like this:
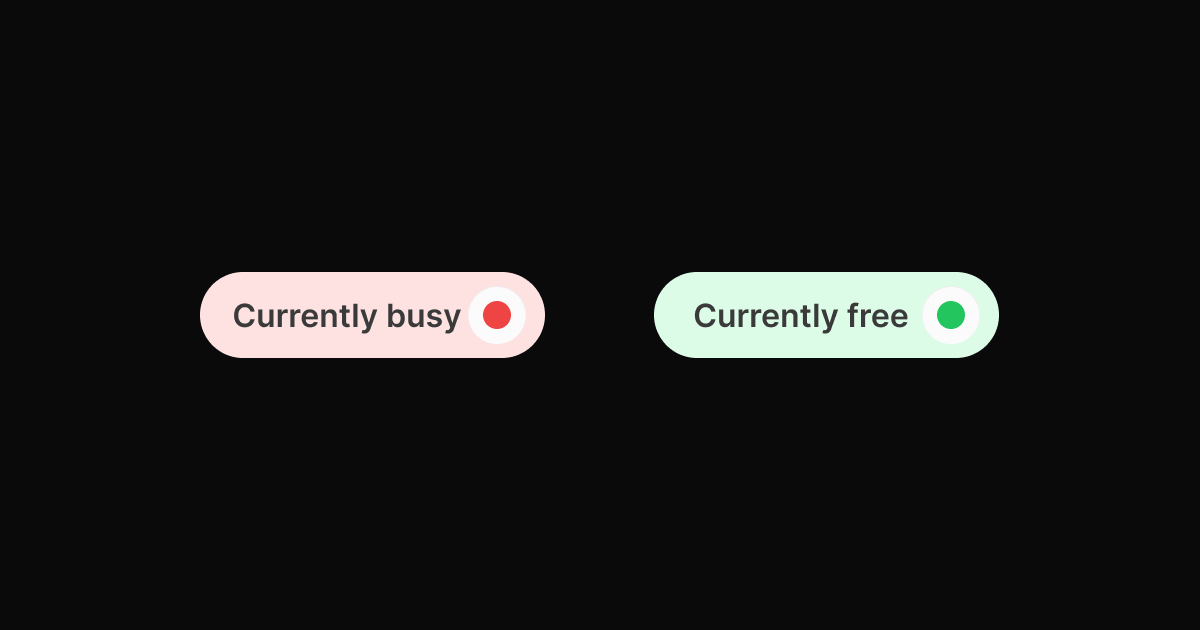